In the previous article (Part 1), we successfully established a custom post type (CPT) specifically designed for FAQs. This streamlined the organization and management of our FAQ content. However, there’s an additional layer of WordPress functionality we can use to further enhance our FAQs: custom fields.
This is part of a multi-part series. Part one shows you how to create a custom post type in WordPress. Part three will show you how to create a custom taxonomy for you custom post type. And finally, part four will walk you through creating a template for the front end.
WordPress Custom Fields Explained
Think of custom fields as specialized compartments within your custom post types. These enable you to store extra information relevant to each entry. Custom fields in WordPress function like labels you attach to provide additional details or categorize them.
I’ll be using custom fields to store a link to a relevant blog post for each FAQ. This integration allows users to transition from a brief FAQ response to a more in-depth explanation on the blog. Ultimately, this should enhance their user experience. I’ll also add a weight system to allow for easy re-organizing.
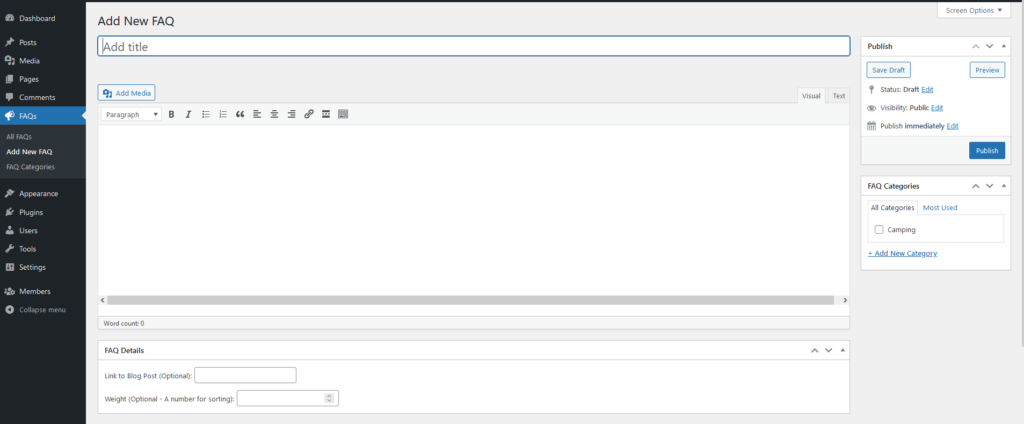
Starting the Code
add_custom_faq_fields
: This function serves as the mastermind behind the operation. It strategically hooks into two WordPress actions: add_meta_boxes
and save_post
. The first action essentially instructs WordPress to execute the create_faq_meta_box
function whenever a meta box (the designated area where our custom fields reside) is added. The second action guarantees that whenever an FAQ post is saved, the save_faq_meta_data
function springs into action to preserve our precious custom field data.
function add_custom_faq_fields() {
add_action( 'add_meta_boxes', 'create_faq_meta_box' );
add_action( 'save_post', 'save_faq_meta_data' );
}
add_action( 'init', 'add_custom_faq_fields' );
Note the final line where we add the action that calls the add_custom_faq_fields() method on initialization.
Building the Meta Box With the create_faq_meta_box
Function
This function creates a dedicated meta box within the WordPress admin area specifically tailored for our FAQ custom post type. Essentially, it creates a designated zone for adding custom field magic to our FAQs. It accepts four arguments:
'faq_meta_box'
: This assigns a unique identifier to our meta box.'FAQ Details'
: This establishes the title displayed at the top of the meta box.'display_faq_meta_box'
: This informs WordPress which function to utilize for rendering the actual content (our custom fields) within the meta box.'faq'
: This ensures that the meta box appears exclusively when editing FAQ CPT entries.
There are also two additional (optional) arguments:
- **
'normal'
&'high'
: These arguments define the placement and priority of the meta box on the edit screen. We’ve opted for a normal priority level and positioned it prominently at the top of the screen for optimal accessibility. This ensures our custom fields are readily visible and easily accessible when editing FAQs.
function create_faq_meta_box() {
add_meta_box( 'faq_meta_box', 'FAQ Details', 'display_faq_meta_box', 'faq', 'normal', 'high' );
}
Rendering the Content With the display_faq_meta_box
Function
This function adds the visual aspects within our meta box. The function grabs existing custom field data for the FAQ you’re editing and then actively builds the HTML structure for those fields. This framework encompasses labels, input fields, and a concealed security nonce (but we’ll address that later). Here’s a simplified breakdown of its functionality:
- Retrieving Existing Data: It retrieves the values of any existing custom field data using the
get_post_meta
function. In our example, we’re fetching the values for two custom fields:faq_link
(link to a blog post) andfaq_weight
(weight for sorting). - Building the HTML Structure: It constructs the HTML framework for our custom fields using fundamental HTML elements such as
<p>
(paragraphs) and<label>
(for field labels). Within the labels, it presents clear descriptions of what each custom field represents (e.g., “Link to Blog Post”). This ensures users understand the purpose of each field and can enter the appropriate information. - Creating Input Fields: It establishes the actual input fields using HTML elements like
<input>
. The specific type of input field (text, number, etc.) hinges on the kind of data you intend to collect. For instance, thefaq_link
field might utilize a text input field, while thefaq_weight
field might employ a number input field. This ensures users can provide data in the most suitable format.
By following these steps, the display_faq_meta_box
function constructs the visual elements within our meta box, allowing users to add custom data to their FAQs.
function display_faq_meta_box( $post ) {
$link = get_post_meta( $post->ID, 'faq_link', true ); // Retrieve existing link value
$weight = get_post_meta( $post->ID, 'faq_weight', true ); // Retrieve existing weight value
// HTML structure for your custom fields with labels and input fields
wp_nonce_field( basename( __FILE__ ), 'faq_nonce' ); // Security nonce
?>
<p>
<label for="faq_link">Link to Blog Post (Optional):</label>
<input type="text" name="faq_link" id="faq_link" value="<?php echo esc_attr( $link ); ?>" />
</p>
<p>
<label for="faq_weight">Weight (Optional - A number for sorting):</label>
<input type="number" name="faq_weight" id="faq_weight" value="<?php echo esc_attr( $weight ); ?>" />
</p>
<?php
}
Saving the Data With the save_faq_meta_data
Function
Now that we’ve explored how the create_faq_meta_box
and display_faq_meta_box
functions craft the meta box and its visual elements, let’s shift our focus to the save_faq_meta_data
function. This function acts as a safeguard, checking and storing the custom field data users enter into the WordPress database.
Here’s a breakdown of its functionality:
- Security Check: This function prioritizes security by implementing a verification process. It checks for the presence of a specific hidden form field (often referred to as a nonce) using
isset
andwp_verify_nonce
. This nonce is a one-time security measure embedded within the meta box by thedisplay_faq_meta_box
function. To prevent security issues, this function verifies the data comes from your real meta box before saving it to the database. - Sanitizing User Input: Once the security check is passed, the function sanitizes the user-submitted data retrieved from the custom fields using functions like
esc_url_raw
(for thefaq_link
) and(int)
(for thefaq_weight
). This sanitization process cleanses the user-entered data to prevent malicious code from sneaking in. It then securely stores the sanitized data in the database. - Updating Meta Data: Finally, after the data is verified and sanitized, the function leverages the
update_post_meta
function to update the corresponding custom field data associated with the specific FAQ post being edited. This essentially stores the custom field data within the WordPress database, making it persistently accessible.
By following these steps, the save_faq_meta_data
function plays a vital role in safeguarding and preserving the custom field data entered by users. It can be retrieved and displayed on your website’s front-end to enhance the user experience.
function save_faq_meta_data( $post_id ) {
// Security check
if ( ! isset( $_POST['faq_nonce'] ) || ! wp_verify_nonce( $_POST['faq_nonce'], basename( __FILE__ ) ) ) {
return $post_id;
}
// Sanitize user input before saving
$link = isset( $_POST['faq_link'] ) ? esc_url_raw( $_POST['faq_link'] ) : '';
$weight = isset( $_POST['faq_weight'] ) ? (int) $_POST['faq_weight'] : '';
// Update meta data
update_post_meta( $post_id, 'faq_link', $link );
update_post_meta( $post_id, 'faq_weight', $weight );
}
Complete Code Example for Adding Custom Fields in WordPress
function add_custom_faq_fields() {
add_action( 'add_meta_boxes', 'create_faq_meta_box' );
add_action( 'save_post', 'save_faq_meta_data' );
}
add_action( 'init', 'add_custom_faq_fields' );
function create_faq_meta_box() {
add_meta_box( 'faq_meta_box', 'FAQ Details', 'display_faq_meta_box', 'faq', 'normal', 'high' );
}
function display_faq_meta_box( $post ) {
$link = get_post_meta( $post->ID, 'faq_link', true ); // Retrieve existing link value
$weight = get_post_meta( $post->ID, 'faq_weight', true ); // Retrieve existing weight value
// HTML structure for your custom fields with labels and input fields
wp_nonce_field( basename( __FILE__ ), 'faq_nonce' ); // Security nonce
?>
<p>
<label for="faq_link">Link to Blog Post (Optional):</label>
<input type="text" name="faq_link" id="faq_link" value="<?php echo esc_attr( $link ); ?>" />
</p>
<p>
<label for="faq_weight">Weight (Optional - A number for sorting):</label>
<input type="number" name="faq_weight" id="faq_weight" value="<?php echo esc_attr( $weight ); ?>" />
</p>
<?php
}
function save_faq_meta_data( $post_id ) {
// Security check
if ( ! isset( $_POST['faq_nonce'] ) || ! wp_verify_nonce( $_POST['faq_nonce'], basename( __FILE__ ) ) ) {
return $post_id;
}
// Sanitize user input before saving
$link = isset( $_POST['faq_link'] ) ? esc_url_raw( $_POST['faq_link'] ) : '';
$weight = isset( $_POST['faq_weight'] ) ? (int) $_POST['faq_weight'] : '';
// Update meta data
update_post_meta( $post_id, 'faq_link', $link );
update_post_meta( $post_id, 'faq_weight', $weight );
}
Conclusion: Adding Custom Fields in WordPress
As we’ve explored, custom fields empower you to significantly enhance your WordPress CPTs. In my case, they provide a flexible and effective way to store additional data specific to each FAQ entry: linking to relevant blog posts for in-depth explanations and assigning weights for sorting purposes. By leveraging the concepts and code we’ve covered, you can add custom fields to any of your CPTs.
Part three of this series will guide you through creating a custom taxonomy for your CPT, allowing for even more organized content management. Stay tuned!